Sample Product Catalog App (Golang)
Introduction
🪄 Dive into the world of Product catelog and see how seamlessly Keploy integrates with Mux and Postgres. Buckle up, it's gonna be a fun ride! 🎢
🛠️ Platform-Specific Requirements for Keploy
Below is a table summarizing the tools needed for both native and Docker installations of Keploy on MacOS, Windows, and Linux:
Operating System | Without Docker | Docker Installation | Prerequisites |
---|---|---|---|
![]() | - Docker Desktop version must be 4.25.2 or above - For running Keploy on MacOS natively, refer to Guide | ||
- Use WSL wsl --install - Windows 10 version 2004 and higher (Build 19041 and higher) or Windows 11 | |||
![]() | Linux kernel 5.15 or higher |
On MacOS and Windows, additional tools are required for Keploy due to the lack of native eBPF support.
Keploy Installation
Quick Installation Using CLI
Let's get started by setting up the Keploy alias with this command:
curl --silent -O -L https://keploy.io/install.sh && source install.sh
You should see something like this:
▓██▓▄
▓▓▓▓██▓█▓▄
████████▓▒
▀▓▓███▄ ▄▄ ▄ ▌
▄▌▌▓▓████▄ ██ ▓█▀ ▄▌▀▄ ▓▓▌▄ ▓█ ▄▌▓▓▌▄ ▌▌ ▓
▓█████████▌▓▓ ██▓█▄ ▓█▄▓▓ ▐█▌ ██ ▓█ █▌ ██ █▌ █▓
▓▓▓▓▀▀▀▀▓▓▓▓▓▓▌ ██ █▓ ▓▌▄▄ ▐█▓▄▓█▀ █▓█ ▀█▄▄█▀ █▓█
▓▌ ▐█▌ █▌
▓
Keploy CLI
Available Commands:
example Example to record and test via keploy
config --generate generate the keploy configuration file
record record the keploy testcases from the API calls
test run the recorded testcases and execute assertions
update Update Keploy
Flags:
--debug Run in debug mode
-h, --help help for keploy
-v, --version version for keploy
Use "keploy [command] --help" for more information about a command.
🎉 Wohoo! You are all set to use Keploy.
Other Installation Methods
Install using Docker
Downloading and running Keploy in Docker
On macOS
Note : Keploy is not supported natively on MacOS, so you can follow the below method to run with docker
-
Open up a terminal window.
-
Create a bridge network in Docker using the following docker network create command:
docker network create keploy-network
- Run the following command to start the Keploy container:
alias keploy="docker run --name keploy-v2 -p 16789:16789 --network keploy-network --privileged --pid=host -v $(pwd):$(pwd) -w $(pwd) -v /sys/fs/cgroup:/sys/fs/cgroup -v /sys/kernel/debug:/sys/kernel/debug -v /sys/fs/bpf:/sys/fs/bpf -v /var/run/docker.sock:/var/run/docker.sock --rm ghcr.io/keploy/keploy"
Downloading and running Keploy in Native
Downloading and running Keploy in Native
Prequisites:
- Linux Kernel version 5.15 or higher
- Run
uname -a
to verify the system architecture. - In case of Windows, use WSL with Ubuntu 20.04 LTS or higher.
Downloading and running Keploy On WSL/Linux AMD
On WSL/Linux AMD
- Open the terminal Session.
- Run the following command to download and install Keploy:
curl --silent --location "https://github.com/keploy/keploy/releases/latest/download/keploy_linux_amd64.tar.gz" | tar xz --overwrite -C /tmp
sudo mkdir -p /usr/local/bin && sudo mv /tmp/keploy /usr/local/bin/keploy
On WSL/Linux ARM
- Open the terminal Session
- Run the following command to download and install Keploy:
curl --silent --location "https://github.com/keploy/keploy/releases/latest/download/keploy_linux_arm64.tar.gz" | tar xz --overwrite -C /tmp
sudo mkdir -p /usr/local/bin && sudo mv /tmp/keploy /usr/local/bin/keploy
Note: Keploy is not supported on MacOS natively.
Setting up the Docker Desktop for WSL 2
- Install Docker Desktop for Windows from here.
When developing on Windows with Docker Desktop and WSL 2, it's crucial to configure Docker Desktop to allow WSL 2 distributions to access the Docker daemon. This setup enables seamless integration between your Windows environment, WSL 2 Linux distros, and Docker.
By default, Docker Desktop may not be configured to work with all WSL 2 distros out of the box. Proper configuration ensures that you can run Docker commands from within your WSL 2 environment, allowing for a more native Linux development experience while leveraging the power of Windows.
This setup is essential for Keploy to function correctly in a WSL 2 environment, as it needs to interact with the Docker daemon to manage containers and networks effectively. For detailed instructions on how to configure
Docker Desktop
for WSL 2, please refer to the official Docker documentation.
Get Started! 🎬
Clone a sample Product Catalog App🧪
git clone https://github.com/keploy/samples-go.git && cd samples-go/mux-sql
go mod download
Installation 📥
There are 2 ways you can run this sample application.
- Using Docker compose : running application as well as Postgres on Docker container
- Using Docker container for Postgres and running application locally
Using Docker Compose 🐳
We will be using Docker compose to run the application as well as Postgres on Docker container.
Lights, Camera, Record! 🎥
Fire up the application and Postgres instance with Keploy. Keep an eye on the two key flags:
-c
: Command to run the app (e.g., docker compose up
).
--container-name
: The container name in the docker-compose.yml
for traffic interception.
Capture the TestCase
keploy record -c "docker compose up" --container-name "muxSqlApp" --build-delay 50
🔥 Challenge time! Generate some test cases. How? Just make some API calls. Postman, Hoppscotch or even curl - take your pick!
Generate a Test Case
curl --request POST \
--url http://localhost:8010/product \
--header 'content-type: application/json' \
--data '{
"name":"Bubbles",
"price": 123
}'
Here's a peek of what you get:
{
"id": 1,
"name": "Bubbles",
"price": 123
}
🎉 Woohoo! With a simple API call, you've crafted a test case with a mock! Dive into the Keploy directory and feast your eyes on the newly minted test-1.yml
and mocks.yml
version: api.keploy.io/v1beta2
kind: Http
name: test-1
spec:
metadata: {}
req:
method: POST
proto_major: 1
proto_minor: 1
url: http://localhost:8010/product
header:
Accept: "*/*"
Content-Length: "46"
Content-Type: application/json
Host: localhost:8010
User-Agent: curl/8.1.2
body: |-
{
"name":"Bubbles",
"price": 123
}
body_type: ""
resp:
status_code: 201
header:
Content-Length: "37"
Content-Type: application/json
Date: Mon, 09 Oct 2023 06:51:16 GMT
body: '{"id":4,"name":"Bubbles","price":123}'
body_type: ""
status_message: ""
proto_major: 0
proto_minor: 0
objects: []
assertions:
noise:
- header.Date
created: 1696834280
this is how mocks.yml
generated would look like:-
version: api.keploy.io/v1beta2
kind: Postgres
name: mocks
spec:
metadata: {}
postgresrequests:
- origin: client
message:
- type: binary
data: AAAAZgADAABleHRyYV9mbG9hdF9kaWdpdHMAMgB1c2VyAHBvc3RncmVzAGRhdGFiYXNlAHBvc3RncmVzAGNsaWVudF9lbmNvZGluZwBVVEY4AGRhdGVzdHlsZQBJU08sIE1EWQAA
postgresresponses:
- origin: server
message:
- type: binary
data: UgAAAAwAAAAF0ykSRQ==
---
version: api.keploy.io/v1beta2
kind: Postgres
name: mocks
spec:
metadata: {}
postgresrequests:
- origin: client
message:
- type: binary
data: cAAAAChtZDU3ZmY0ZTZhZGEzMThlZDJiYWM5ODQyY2YwNmEyODE2MwA=
postgresresponses:
- origin: server
message:
- type: binary
data: UgAAAAgAAAAAUwAAABZhcHBsaWNhdGlvbl9uYW1lAABTAAAAGWNsaWVudF9lbmNvZGluZwBVVEY4AFMAAAAXRGF0ZVN0eWxlAElTTywgTURZAFMAAAAZaW50ZWdlcl9kYXRldGltZXMAb24AUwAAABtJbnRlcnZhbFN0eWxlAHBvc3RncmVzAFMAAAAUaXNfc3VwZXJ1c2VyAG9uAFMAAAAZc2VydmVyX2VuY29kaW5nAFVURjgAUwAAADFzZXJ2ZXJfdmVyc2lvbgAxMC41IChEZWJpYW4gMTAuNS0yLnBnZGc5MCsxKQBTAAAAI3Nlc3Npb25fYXV0aG9yaXphdGlvbgBwb3N0Z3JlcwBTAAAAI3N0YW5kYXJkX2NvbmZvcm1pbmdfc3RyaW5ncwBvbgBTAAAAEVRpbWVab25lAFVUQwBLAAAADAAAAB6JC1lnWgAAAAVJ
---
version: api.keploy.io/v1beta2
kind: Postgres
name: mocks
spec:
metadata: {}
postgresrequests:
- origin: client
message:
- type: binary
data: UAAAAEUASU5TRVJUIElOVE8gcHJvZHVjdHMobmFtZSwgcHJpY2UpIFZBTFVFUygkMSwgJDIpIFJFVFVSTklORyBpZAAAAEQAAAAGUwBTAAAABA==
postgresresponses:
- origin: server
message:
- type: binary
data: MQAAAAR0AAAADgACAAAAGQAABqRUAAAAGwABaWQAAABAAgABAAAAFwAE/////wAAWgAAAAVJ
Fetch Product from Catalog
curl --request GET \ --url http://localhost:8010/products
Or just type http://localhost:8010/products
in your browser. Your choice!
Spotted the new test and mock files in your project? High five! 🙌
Run Tests
Time to put things to the test 🧪
keploy test -c "docker compose up" --container-name "muxSqlApp" --build-delay 50 --delay 10
The
--delay
flag? Oh, that's just giving your app a little breather (in seconds) before the test cases come knocking.
Final thoughts? Dive deeper! Try different API calls, tweak the DB response in the mocks.yml
, or fiddle with the request or response in test-x.yml
. Run the tests again and see the magic unfold!✨👩💻👨💻✨
Wrapping it up 🎉
Congrats on the journey so far! You've seen Keploy's power, flexed your coding muscles, and had a bit of fun too! Now, go out there and keep exploring, innovating, and creating! Remember, with the right tools and a sprinkle of fun, anything's possible.😊🚀
Happy coding! ✨👩💻👨💻✨
**********************************___**********************************
Running App Locally on Linux/WSL 🐧
We'll be running our sample application right on Linux, but just to make things a tad more thrilling, we'll have the database (Postgres) chill on Docker. Ready? Let's get the party started!🎉
First things first, update the First things first, update the postgres host on line 10 in main.go, update the host to localhost
.
🍃 Kickstart PostgresDB
Let's breathe life into your Postgres container. A simple spell should do the trick:
docker compose up postgres
📼 Roll the Tape - Recording Time!
Ready, set, record! Here's how:
sudo -E env PATH=$PATH keploy record -c "go run main.go app.go"
Keep an eye out for the -c
flag! It's the command charm to run the app. Whether you're using go run main.go app.go
or the binary path like ./test-app-product-catelog
, it's your call.
Alright, magician! With the app alive and kicking, let's weave some test cases. The spell? Making some API calls! Postman, Hoppscotch, or the classic curl - pick your wand.
Generate a Test Case
✨ A pinch of URL magic:
curl --request POST \
--url http://localhost:8010/product \
--header 'content-type: application/json' \
--data '{
"name":"Bubbles",
"price": 123
}'
And... voila! A Product entry appears:
{
"id": 1,
"name": "Bubbles",
"price": 123
}
Give yourself a pat on the back! With that simple spell, you've conjured up a test case with a mock! Explore the Keploy directory and you'll discover your handiwork in test-1.yml
and mocks.yml
.
version: api.keploy.io/v1beta2
kind: Http
name: test-1
spec:
metadata: {}
req:
method: POST
proto_major: 1
proto_minor: 1
url: http://localhost:8010/product
header:
Accept: "*/*"
Content-Length: "46"
Content-Type: application/json
Host: localhost:8010
User-Agent: curl/8.1.2
body: |-
{
"name":"Bubbles",
"price": 123
}
body_type: ""
resp:
status_code: 201
header:
Content-Length: "37"
Content-Type: application/json
Date: Mon, 09 Oct 2023 06:51:16 GMT
body: '{"id":4,"name":"Bubbles","price":123}'
body_type: ""
status_message: ""
proto_major: 0
proto_minor: 0
objects: []
assertions:
noise:
- header.Date
created: 1696834280
this is how mocks.yml
generated would look like:-
version: api.keploy.io/v1beta2
kind: Postgres
name: mocks
spec:
metadata: {}
postgresrequests:
- origin: client
message:
- type: binary
data: AAAAZgADAABleHRyYV9mbG9hdF9kaWdpdHMAMgB1c2VyAHBvc3RncmVzAGRhdGFiYXNlAHBvc3RncmVzAGNsaWVudF9lbmNvZGluZwBVVEY4AGRhdGVzdHlsZQBJU08sIE1EWQAA
postgresresponses:
- origin: server
message:
- type: binary
data: UgAAAAwAAAAF0ykSRQ==
---
version: api.keploy.io/v1beta2
kind: Postgres
name: mocks
spec:
metadata: {}
postgresrequests:
- origin: client
message:
- type: binary
data: cAAAAChtZDU3ZmY0ZTZhZGEzMThlZDJiYWM5ODQyY2YwNmEyODE2MwA=
postgresresponses:
- origin: server
message:
- type: binary
data: UgAAAAgAAAAAUwAAABZhcHBsaWNhdGlvbl9uYW1lAABTAAAAGWNsaWVudF9lbmNvZGluZwBVVEY4AFMAAAAXRGF0ZVN0eWxlAElTTywgTURZAFMAAAAZaW50ZWdlcl9kYXRldGltZXMAb24AUwAAABtJbnRlcnZhbFN0eWxlAHBvc3RncmVzAFMAAAAUaXNfc3VwZXJ1c2VyAG9uAFMAAAAZc2VydmVyX2VuY29kaW5nAFVURjgAUwAAADFzZXJ2ZXJfdmVyc2lvbgAxMC41IChEZWJpYW4gMTAuNS0yLnBnZGc5MCsxKQBTAAAAI3Nlc3Npb25fYXV0aG9yaXphdGlvbgBwb3N0Z3JlcwBTAAAAI3N0YW5kYXJkX2NvbmZvcm1pbmdfc3RyaW5ncwBvbgBTAAAAEVRpbWVab25lAFVUQwBLAAAADAAAAB6JC1lnWgAAAAVJ
---
version: api.keploy.io/v1beta2
kind: Postgres
name: mocks
spec:
metadata: {}
postgresrequests:
- origin: client
message:
- type: binary
data: UAAAAEUASU5TRVJUIElOVE8gcHJvZHVjdHMobmFtZSwgcHJpY2UpIFZBTFVFUygkMSwgJDIpIFJFVFVSTklORyBpZAAAAEQAAAAGUwBTAAAABA==
postgresresponses:
- origin: server
message:
- type: binary
data: MQAAAAR0AAAADgACAAAAGQAABqRUAAAAGwABaWQAAABAAgABAAAAFwAE/////wAAWgAAAAVJ
Now, the real fun begins. Let's weave more spells!
Fetch Product from Catalog
🚀 Follow the URL road...!
curl --request GET \ --url http://localhost:8010/products
Or simply wander over to your browser and visit http://localhost:8010/products
.
Did you spot the new test and mock scrolls in your project library? Awesome! 👏
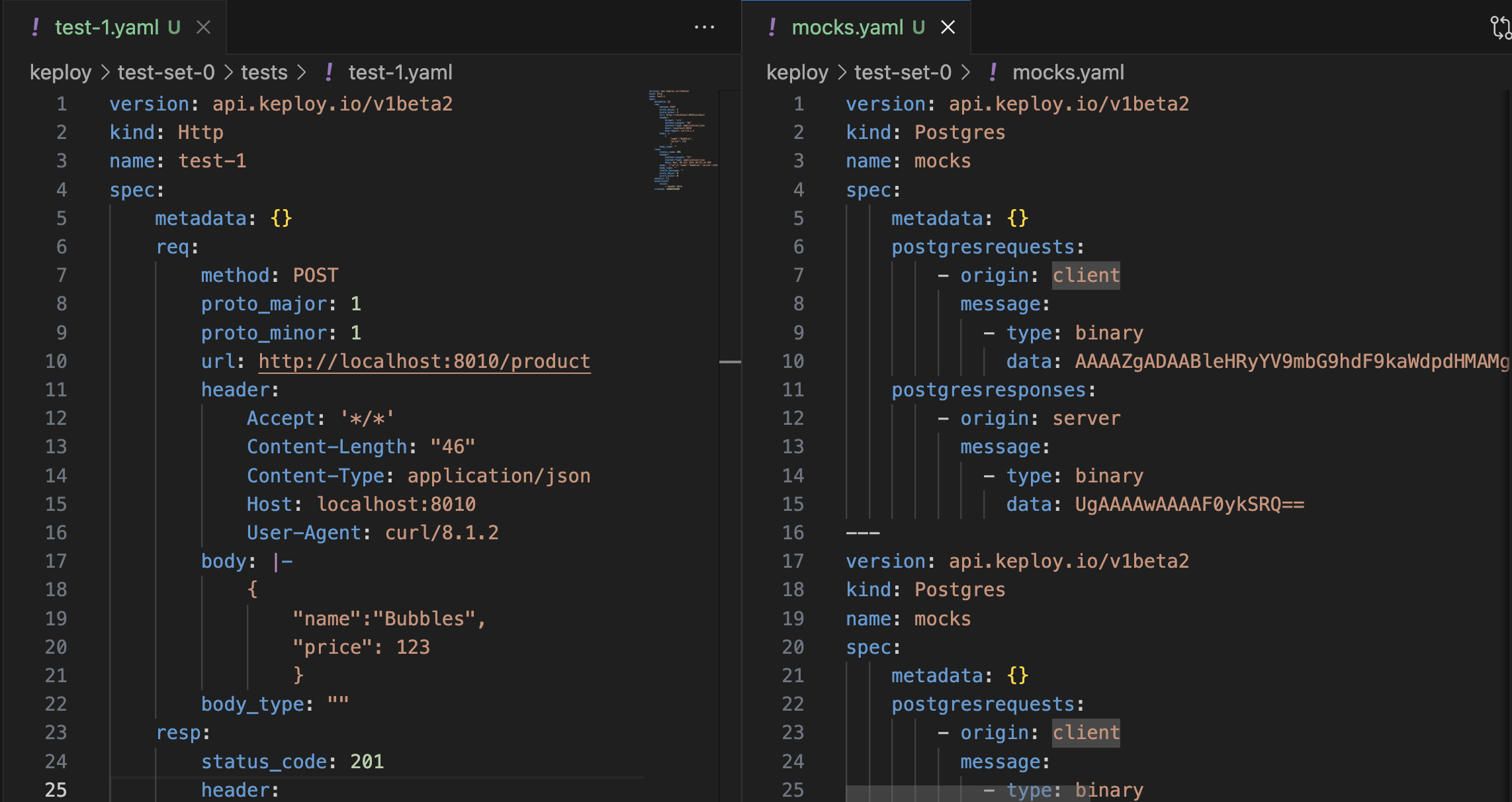
Run Tests 🏁
Ready to put your spells to the test?
sudo -E env PATH=$PATH keploy test -c "go run main.go app.go" --delay 10
Final thoughts? Dive deeper! Try different API calls, tweak the DB response in the mocks.yml
, or fiddle with the request or response in test-x.yml
. Run the tests again and see the magic unfold! ✨👩💻👨💻✨
Wrapping it up 🎉
Congrats on the journey so far! You've seen Keploy's power, flexed your coding muscles, and had a bit of fun too! Now, go out there and keep exploring, innovating, and creating! Remember, with the right tools and a sprinkle of fun, anything's possible. 😊🚀
Hope this helps you out, if you still have any questions, reach out to us .
Contact Us
If you have any questions or need help, please feel free to reach out to us at hello@keploy.io or reach out us on
or open a discussion on